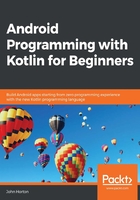
Writing our first Kotlin code
So, we now know the code that will output to logcat or the user's screen. However, where do we put the code? To answer this question, we need to understand that the onCreate
function in MainActivity.kt
executes as the app is preparing to be shown to the user. So, if we put our code at the end of this function, it will run just as the user sees it; that sounds good.
Tip
We know that to execute the code in a function, we need to call it. We have wired our buttons up to call a couple of functions, such as topClick
and bottomClick
. Soon, we will write these functions. But who or what is calling onCreate
? The answer to this mystery is that Android itself calls onCreate
in response to the user clicking on the app icon to run the app. In Chapter 6, The Android Lifecycle, we will look deeper, and it will be clear what exactly the code executes and when. You don't need to completely comprehend this now; I just wanted to give you an overview of what was going on.
Let's quickly try this out; switch to the MainActivity.kt
tab in Android Studio.
We know that the onCreate
function is called just before the app starts for real. Let's copy and paste some code into the onCreate
function of our Hello World app and see what happens when we run it.
Adding message code to the onCreate function
Find the closing curly brace (}
) of the onCreate
function and add the highlighted code shown in the following code block. In the code, I haven't shown the complete content of the onCreate
function, but instead, I have used …
to indicate a number of lines of code not being shown. The important thing is to place the new code (shown in full) right at the end, but before the closing curly brace (}
):
override fun onCreate(savedInstanceState: Bundle?) { … … … // Your code goes here Toast.makeText(this, "Can you see me?", Toast.LENGTH_SHORT).show() Log.i("info", "Done creating the app") }
Notice that the instances of Toast
and Log
are highlighted in red in Android Studio. They are errors. We know that Toast
and Log
are classes and that classes are containers for code.
The problem is that Android Studio doesn't know about them until we tell it. We must add an import
directive for each class. Fortunately, this is semi-automatic.
Left-click on Toast
; now hold the Alt key and tap Enter. You need to do this step twice, once for Toast
, and once for Log
. Android Studio adds the import
directives at the top of the code with our other imports, and the errors are gone.
Tip
Alt + Enter is just one of many useful keyboard shortcuts. The following is a keyboard shortcut reference for Android Studio. More specifically, it is for the IntelliJ Idea IDE, which Android Studio is based on. Bookmark this web page; it will be invaluable over the course of this book: http://www.jetbrains.com/idea/docs/IntelliJIDEA_ReferenceCard.pdf.
Scroll to the top of MainActivity.kt
and look at the added import
directives. Here they are for your convenience:
import android.util.Log import android.widget.Toast
Run the app in the usual way and look at the output in the logcat window.
Examining the output
The following is a screenshot of the output in the logcat window:
Looking at the logcat, you can see that our message – Done creating the app – was output, although it is mixed up amongst other system messages that we are currently not interested in. If you watched the emulator when the app first starts, you will also see the neat pop-up message that the user will see:
You might be wondering why the messages were output at the time that they were. The simple answer is that the onCreate
function is called just before the app starts to respond to the user. It is common practice amongst Android developers to put code in this function to get their apps set up and ready for user input.
Now, we will go a step further and write our own functions that are called by our UI buttons. We will place similar Log
and Toast
messages inside them.
Writing our own Kotlin functions
Let's go straight on to writing our first Kotlin functions with some more Log
and Toast
messages inside them.
Tip
Now will be a good time, if you haven't done so already, to get the download bundle that contains all the code files. You can view the completed code for each chapter. For example, the completed code for this chapter can be found in the Chapter02
folder. I have further subdivided the Chapter02
folder into kotlin
and res
folders (for Kotlin and resource files). In chapters with more than one project, I will divide the folders further to include the project name. You should view these files in a text editor. My favorite is Notepad++, a free download from https://notepad-plus-plus.org/download/. Code viewed in a text editor is easier to read than directly from the book, especially the paperback version, and even more so where the lines of code are long. The text editor is also a great way to select sections of the code to copy and paste into Android Studio. You could open the code in Android Studio, but then you risk mixing up my code with the autogenerated code of Android Studio.
Identify the closing curly brace (}
) of the MainActivity
class.
Tip
Note that you are looking for the end of the entire class, not the end of the onCreate
function, as in the previous section. Take your time to identify the new code and where it goes among the existing code.
Inside that curly brace, enter the following code that is highlighted:
override fun onCreate(savedInstanceState: Bundle?) { … … … … } … … … fun topClick(v: View) { Toast.makeText(this, "Top button clicked", Toast.LENGTH_SHORT).show() Log.i("info", "The user clicked the top button") } fun bottomClick(v: View) { Toast.makeText(this, "Bottom button clicked", Toast.LENGTH_SHORT).show() Log.i("info", "The user clicked the bottom button") } } // This is the end of the class
Notice that the two instances of View
are in red, indicating an error. Simply use the Alt + Enter keyboard combination to import the View
class and remove the errors.
Deploy the app to a real device or emulator in the usual way and start tapping the buttons so that we can observe the output.
Examining the output
At last, our app does something! We can see that the function names we defined in the button onClick
attribute are indeed called when the buttons are clicked on; the appropriate messages are added to the logcat window; and the appropriate Toast
messages are shown to the user.
Admittedly, we still don't understand why Toast
and Log
really work, nor do we fully comprehend the (v: View)
parts of our function's syntax, or the rest of the autogenerated code. This will become clear as we progress. As stated previously, in Chapter 10, Object-Oriented Programming, we will take a deep dive into the world of classes and, in Chapter 9, Kotlin Functions, we will master the rest of the syntax associated with functions.
Take a look at the logcat output; you can see that a log entry was made from the onCreate
function just as before, as well as from the two functions that we wrote ourselves, each time you clicked on a button. In the following screenshot, you can see that I clicked on each button three times:
As you are now familiar with where to find the logcat window, in future, I will present the logcat output as trimmed text as it is clearer to read:
The user clicked the top button The user clicked the top button The user clicked the top button The user clicked the bottom button The user clicked the bottom button The user clicked the bottom button
In the following screenshot, you can see that the top button has been clicked on and that the topClick
function was called, triggering the pop-up Toast
message:
Throughout this book, we will regularly output to logcat, so that we can see what is going on behind the UI of our apps. Toast
messages are more for notifying the user that something has occurred. This might be a download that has completed, a new email has arrived, or some other occurrence that needs their attention.