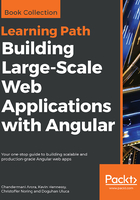
Workout and exercise list components
First, open the workouts.component.ts file in the trainer/src/app/workout-builder/workouts folder and update the imports as follows:
import { Component, OnInit } from '@angular/core';
import { Router } from '@angular/router';
import { WorkoutPlan } from '../../core/model';
import { WorkoutService } from '../../core/workout.service';;
This new code imports the Angular Router as well as WorkoutService and the WorkoutPlan type.
Next, replace the class definition with the following code:
export class WorkoutsComponent implements OnInit { workoutList:Array<WorkoutPlan> = []; constructor( public router:Router, public workoutService:WorkoutService) {} ngOnInit() { this.workoutList = this.workoutService.getWorkouts(); } onSelect(workout: WorkoutPlan) { this.router.navigate( ['./builder/workout', workout.name] ); } }
This code adds a constructor into which we are injecting the Router and the WorkoutService. The ngOnInit method then calls the getWorkouts method on the WorkoutService and populates a workoutList array with a list of WorkoutPlans returned from that method call. We'll use that workoutList array to populate the list of workout plans that will display in the Workouts component's view.
You'll notice that we are putting the code for calling WorkoutService into the ngOnInit method. We want to avoid placing this code in the constructor. Eventually, we will be replacing the in-memory store that this service uses with a call to an external data store and we do not want the instantiation of our component to be affected by this call. Adding these method calls to the constructor would also complicate testing the component.
To avoid such unintended side effects, we instead place the code in the ngOnInit method. This method implements one of Angular's lifecycle hooks, OnInit, which Angular calls after creating an instance of the service. This way, we rely on Angular to call this method in a predictable way that does not affect the instantiation of the component.
Next, we'll make almost identical changes to the Exercises component. As with the Workouts component, this code injects the workout service into our component. This time, we then use the workout service to retrieve the exercises.