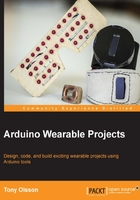
The accelerometer, compass, and gyroscope
In the following example, we will try out an accelerometer, which is a device used for sensing g-force. The accelerometer can sense movement in different directions. Normally, there are two types of accelerometer: the 2 axes and 3 axes. The 2 axes measures in two directions, left to right and front to back, and these directions are often named as the x axis and y axis. The 3 axes accelerometer also measures in a z axis, which is up and down. The accelerometer measures movement relative to its own position, which means that when you move it, it measures the g-force in the direction you are moving it.
The sensor used in this example is the FLORA accelerometer/compass/gyroscope, which is actually three sensors in one. Besides the accelerometer, it holds a compass, known as a magnetometer, which senses where the strongest magnetic field is coming from. If there are no other magnets near by, the strongest magnetic field comes from the Earth's north pole.
The third sensor is the gyroscope, which is a device that can sense rate of orientation on a spin axis through external torque. This basically means that the gyroscope can sense in which direction you are turning, compared to the accelerometer that senses which direction you are tilting the sensor.
In previous examples, we have been using serial communication to communicate with our sensors, but in the case of the FLORA accelerometer/compass/gyro, we will be using a different communication protocol called I2C. The I2C is a protocol developed by Philips, and is used to connect low-speed devices to microprocessors, among other things. It uses a master-slave system, which involves one device acting as the master and all the other devices connected to the communication channel acting as slaves. The master can talk to all of the slaves, but the slaves can only talk to the master. I2C is a two-wire protocol where you can hook up multiple slaves to the same communication lines in any order you want, since all slaves are given a unique ID to keep track of them. Keeping track of the communication is the master job so no more than one slave can communicate at the time. In the case of this example, we only have two devices, the FLORA, which will be acting as a master, and the accelerometer/compass/gyro sensor, acting as slaves. Using I2C, we can communicate with all three sensors without connecting them on separate communication channels.
The FLORA accelerometer/compass/gyro is based on a chip called LSM9DS0, which by default is a bit tricky to communicate with. There are many breakout boards based on the LSM9DS0 available, and most of them are supplied with Arduino libraries, even the FLORA one. On the following GitHub link, https://github.com/adafruit/Adafruit_LSM9DS0_Library you can find the Adafruit LSM9DS0
library written by Kevin Towsend, which is an excellent library that simplifies the communication with the LSM9DS0
. Just press the Download ZIP button on the GitHub repository and the library will start downloading.
As I mentioned before, libraries contain code that is usually too long and complicated to copy. In most cases, the code is used for different functionalities, and does not need to be changed. In order to make life easier for others to use their code, some kind souls have made them into libraries so that they can easily be shared.
Once the LSM9DS0
library is downloaded, you need to unzip the folder and rename it to Adafruit_LSM9DS0.
then place it into your libraries folder, inside you Arduino folder, by navigating to Arduino | libraries. If this is the first time you are installing a library, this folder might be missing, and in that case, you just create a folder inside the Arduino folder called libraries
. To find out where your libraries folder is located, you can check in the Arduino IDE by navigating to Arduino | preferences.
This will open up a new window that shows the full path to your libraries folder.
The LSM9DS0
library is dependent on another library by Adafruit, which you also need to install, and you can find it on the following link:
https://github.com/adafruit/Adafruit_Sensor.
Install it as you did with the LSM9DS0
library and rename it as Adafruit_Sensor
. When the libraries are installing, we can carry on with hooking up our sensor to the FLORA board, as shown in Figure 2.10:

Figure 2.10: Connecting the sensor to the FLORA board
The first connection that needs to be made is the 3.3 V, which connects to the 3.3 V pin on the FLORA board. Then connect SDA, SCL, and GND to the same pins with the same name on the FLORA board. Once all the connections have been made, you can enter the following code into the IDE and upload it to the FLORA board:
/*Import the necessary libraries. Wire.h and SPI.h are included in the IDE and does not need to be downloaded*/ #include <Wire.h> #include <SPI.h> #include <Adafruit_LSM9DS0.h> #include <Adafruit_Sensor.h> // set up i2c Adafruit_LSM9DS0 lsm = Adafruit_LSM9DS0(); void setupSensor() { /*Set the accelerometer range where the range can be changed to 4G,8G or 16G */ lsm.setupAccel(lsm.LSM9DS0_ACCELRANGE_2G); //lsm.setupAccel(lsm.LSM9DS0_ACCELRANGE_4G); //lsm.setupAccel(lsm.LSM9DS0_ACCELRANGE_6G); //lsm.setupAccel(lsm.LSM9DS0_ACCELRANGE_8G); //lsm.setupAccel(lsm.LSM9DS0_ACCELRANGE_16G); } void setup() { //Wait for communication to start while (!Serial); //Set the baud rate Serial.begin(9600); //Print message to serial monitor Serial.println("Starting communication"); // Try to initialize and warn if we couldn't detect the chip if (!lsm.begin()) { Serial.println("Something went wrong, check you connections"); while (1); } Serial.println("Connection established"); Serial.println(""); Serial.println(""); } void loop() { //Get data from the sensor lsm.read(); //Print the accelrometer sensor data to the serial monitor Serial.print("Accel X: "); Serial.print((int)lsm.accelData.x); Serial.print(" "); Serial.print("Y: "); Serial.print((int)lsm.accelData.y); Serial.print(" "); Serial.print("Z: "); Serial.println((int)lsm.accelData.z); Serial.print(" "); delay(200); }
This code example initializes the accelerometer and prints the data from the sensor back to the serial monitor. Don't forget to open the monitor in order to see the data flow. If the sensor is left untouched, you will find that the sensor data does not change that much. In the case of my sensor, the values on all three axes ranged from +30
to -30
as some noise is expected. When I start tilting the sensor to the left, the value starts to increase on the x axis, and if I tilt it to the right, the x axis value starts to decrease. The value range on your sensor might be different, so try it out yourself by tilting the sensor in different directions while looking at the serial monitor to get a sense of you sensor range. Accelerometers can only sense movement in 180 degrees, so once your flip it to 180 degrees, the value starts over for the corresponding value on the other side.
In the next code example, we added the functionality of both the compass and gyroscope along side the accelerometer code in order to show the sensors full functionality.
//Import the necessary libraries #include <Wire.h> #include <SPI.h> #include <Adafruit_LSM9DS0.h> #include <Adafruit_Sensor.h> // set up i2c Adafruit_LSM9DS0 lsm = Adafruit_LSM9DS0(); void setupSensor() { /*Set the accelerometer range where the range can be changed to 4G,8G or 16G*/ lsm.setupAccel(lsm.LSM9DS0_ACCELRANGE_2G); //Set the magnetometer sensitivity where the range can be changed to 4GAUSS, 8GAUSS or 12GAUSS lsm.setupMag(lsm.LSM9DS0_MAGGAIN_2GAUSS); /*Setup the gyroscope sensitivity where the range can be changed 500DPS or 2000DPS*/ lsm.setupGyro(lsm.LSM9DS0_GYROSCALE_245DPS); } void setup() { //Wait for communication to start while (!Serial); //Set the baud rate Serial.begin(9600); //Print message to serial monitor Serial.println("Starting communication"); // Try to initialize and warn if we couldn't detect the chip if (!lsm.begin()) { Serial.println("Something went wrong, check you connections"); while (1); } Serial.println("Connection established"); Serial.println(""); Serial.println(""); } void loop() { //Get data from the sensors lsm.read(); //Print the accelrometer sensor data to the serial monitor Serial.print("Accel X: "); Serial.print((int)lsm.accelData.x); Serial.print(" "); Serial.print("Y: "); Serial.print((int)lsm.accelData.y); Serial.print(" "); Serial.print("Z: "); Serial.println((int)lsm.accelData.z); Serial.print(" "); //Print the compass sensor data to the serial monitor Serial.print("Compass X: "); Serial.print((int)lsm.magData.x); Serial.print(" "); Serial.print("Y: "); Serial.print((int)lsm.magData.y); Serial.print(" "); Serial.print("Z: "); Serial.println((int)lsm.magData.z); Serial.print(" "); //Print the gyro sensor data to the serial monitor Serial.print("Gyro X: "); Serial.print((int)lsm.gyroData.x); Serial.print(" "); Serial.print("Y: "); Serial.print((int)lsm.gyroData.y); Serial.print(" "); Serial.print("Z: "); Serial.println((int)lsm.gyroData.z); Serial.println(" "); //wait for a bit delay(200); }
With the help of the preceding code, we will now be able to show the sensors full functionality.