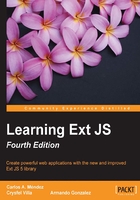
Loading classes on demand
When we develop large applications, performance is really important. We should only load the scripts we need; this means that if we have many modules in our application, we should separate them into packages so we would be able to load them inpidually.
Ext JS, since version 4, allows us to dynamically load classes and files when we need them, also we can configure dependencies in each class and the Ext
library will load them for us.
You need to understand that using the loader is great for development, that way we can easily debug the code because the loader includes all the classes one by one. However, it's not recommended to load all the Ext classes in production environments. We should create packages of classes and then load them when needed, but not class by class.
In order to use the loader system, we need to follow some conventions when defining our class.
- Define only one class per file.
- The name of the class should match the name of the JavaScript file.
- The namespace of the class should match the folder structure. For example, if we define a class
MyApp.customers.controller.Main
, we should have theMain.js
file in theMyApp/customers/controller
path.
Enabling the loader
The loader system is enabled or disabled depending on the Ext
file that we import to our HTML file. If we import the ext-all
or ext-all-debug
file inside the extjs/build
folder, the loader is disabled because all the classes in the Ext
library are loaded already. If we import the ext-all
and ext-all-debug
files inside the extjs
folder, the loader is enabled because only the core classes in the Ext
library are loaded.
If we need to enable the loader, we should do the following at the beginning of the JS file:
Ext.Loader.setConfig({ enabled: true });
The previous code will allow us to load the classes when we need them. Also there's a preprocessor that loads all the dependencies for the given class if they don't exist.
In order to start loading classes, we need to set up the paths where the classes are, and we can do that in two different ways. We can use the setConfig
method to define a paths
property as follows:
Ext.Loader.setConfig({ enabled:true, paths:{ MyApp:'appcode' } });
The paths
property receives an object containing the root namespace of our application and the folder where all the classes are located in this namespace. So in the previous code when we refer to Myapp
, Ext JS will look inside the appcode/
folder. Remember that we can add as many paths or location references as needed.
Once we have enabled and configured the loader correctly, we can start loading our classes using the require
method:
Ext.require([ 'MyApp.Constants', 'MyApp.samples.demoClass' ]);
The require
method creates a script tag behind the scenes. After all the required files are loaded, the onReady
event is fired. Inside the callback, we can use all the loaded classes.
If we try to load the classes after the require
call, we'll get an error because the class won't exist until it's downloaded and created. This is why we need to set the onReady
callback and wait until everything is ready to be used.
In this case, open the loader_01.html
file and check that the file has the correct paths (that script tags are correct) to the ext.js
file instead of ext-all.js
, and run the file in the browser. If you look at the Network traffic tab in the development tools, you will notice the files that were only loaded, which in fact are a few classes (only the classes that Ext JS really need to run the code). Also, the speed of execution of these classes was faster than the previous code samples when we were loading the complete ext-all.js
file located in the build
folder.