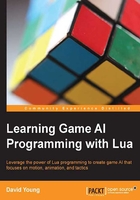
Creating an agent Lua script
To start creating an agent, we need to create another Lua script that implements the Agent_Cleanup
, Agent_HandleEvent
, Agent_Initialize
, and Agent_Update
functions:
Create the Lua file as follows:
src/my_sandbox/script/Agent.lua
Agent.lua
:
function Agent_Cleanup(agent) end function Agent_HandleEvent(agent, event) end function Agent_Initialize(agent) end function Agent_Update(agent, deltaTimeInMillis) end
Now that we have a basic agent script, we can create an instance of the agent within the sandbox. Modify the initialization of the sandbox in order to create your AI agent with the Sandbox.CreateAgent
function.
Tip
Remember that each AI agent runs within its own Lua virtual machine (VM). Even though a separate VM is running the agent logic, you can still access and modify properties of an agent from the sandbox Lua script, as the C++ code manages agent data.
Modify the initialization of the sandbox in order to create your AI agent with the Sandbox.CreateAgent
function.
Sandbox.lua
:
function Sandbox_Initialize(sandbox) ... Sandbox.CreateAgent(sandbox, "Agent.lua"); end
Creating a visual representation
Now that you have an agent running within the sandbox, we need to create a visual representation so that we can see the agent. This time, we use the Core.
CreateCapsule
function to procedurally generate a capsule mesh and attach the mesh to the agent itself. Passing the agent to Core.CreateCapsule
will attach the Ogre mesh directly to the agent and automatically update the position and rotation of the capsule as the agent moves.
We only need to create a visual representation compared to a Sandbox.CreateObject
object, as agents are already simulated within the physics simulation with a capsule representation:
Create the Lua file as follows:
src/my_sandbox/script/AgentUtilities.lua
AgentUtilities.lua
:
function AgentUtilities_CreateAgentRepresentation( agent, height, radius) -- Capsule height and radius in meters. local capsule = Core.CreateCapsule(agent, height, radius); Core.SetMaterial(capsule, "Ground2"); end
Agent.lua
:
function Agent_Initialize(agent) AgentUtilities_CreateAgentRepresentation( agent, agent:GetHeight(), agent:GetRadius()); end
Running the sandbox now will show our agent's visual representation, a capsule using the same Ogre Ground2
material.

A basic capsule representation of our agents
Updating an agent position
To start moving an agent around directly, we can set the agent's position. As agents are simulated within the physics simulation, they will fall to the ground if they are positioned in the air or will be pushed to the top of the ground plane if placed below:
-- Position in meters. local position = Vector.new( xCoordinate, yCoordinate, zCoordinate); Agent.SetPosition(agent, position);
Updating an agent orientation
Changing the orientation of an agent is similar to setting a position vector, except that a forward vector must be provided. As the sandbox simulates humanoid agents, the physics simulation locks the orientation of the agent to force agents upright. When setting a forward vector of an agent, the sandbox assumes that the y axis is considered the up axis within the sandbox:
local forwardDirection = Vector.new( xDirection, 0, zDirection); Agent.SetForward(agent, forwardDirection);