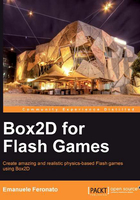
Your first simulation – a ball falling on the floor
We are starting with an easy task, the simplest of all physics simulations: a ball falling on the floor. Anyway, although it's just another falling ball, being your first simulation this will be quite an achievement.
Let's see what we have in our simulation:
- A world with a gravity
- A ball that should react to world forces (such as gravity)
- A floor that shouldn't react to world forces
- Some kind of materials, as we expect the ball to bounce on the floor
You are already able to configure a world with gravity, so we are going to start with the creation of the ball.
- It doesn't matter if we are creating a sphere or a polygon, the first step to create a body is:
var bodyDef:b2BodyDef=new b2BodyDef();
b2BodyDef
is the body definition, which will hold all data needed to create our rigid body. - Now it's time to place the body somewhere in the world. As we are working on a 640 x 480 size, we'll place the ball in the top-center position of the stage, let's say at
(320,30)
, shown as follows:bodyDef.position.Set(10.66,1);
The
position
property obviously sets the body's position in the world, but I am sure you are wondering why I told you that I was going to place the body at(320,30)
and instead placed it at(10.66,1)
. It's a matter of units of measurement. While Flash works with pixels, Box2D tries to simulate the real world and works with meters. There isn't a general rule for converting pixels to meters, but we can say that the following equation works well:meters = pixels * 30
So, if we define a variable to help us with the conversion from meters to pixels, we can use pixels rather than meters when dealing with the Box2D World. This is more intuitive for all of us who are used to thinking in pixels when making Flash games.
- Open the class you have created in the first chapter and change it in the following way:
package { import flash.display.Sprite; import flash.events.Event; import Box2D.Dynamics.*; import Box2D.Collision.*; import Box2D.Collision.Shapes.*; import Box2D.Common.Math.*; public class Main extends Sprite { private var world:b2World; private var worldScale:Number=30; public function Main() { world=new b2World(new b2Vec2(0,9.81),true); var bodyDef:b2BodyDef=new b2BodyDef(); bodyDef.position.Set(320/worldScale,30/worldScale); addEventListener(Event.ENTER_FRAME,updateWorld); } private function updateWorld(e:Event):void { world.Step(1/30,10,10); world.ClearForces(); } } }
Also, notice how I created the world and called the
Step
method. It's just to save some lines of code.
Once you're done with the creation of a body definition, it's time to give it a shape.